Navigating the Minefield of Null Pointer Exceptions
In the world of software development, few errors are as universally dreaded and as common as the null pointer exception (NPE). This sneaky bug can cause applications to crash unexpectedly and can be a major source of frustration for developers.
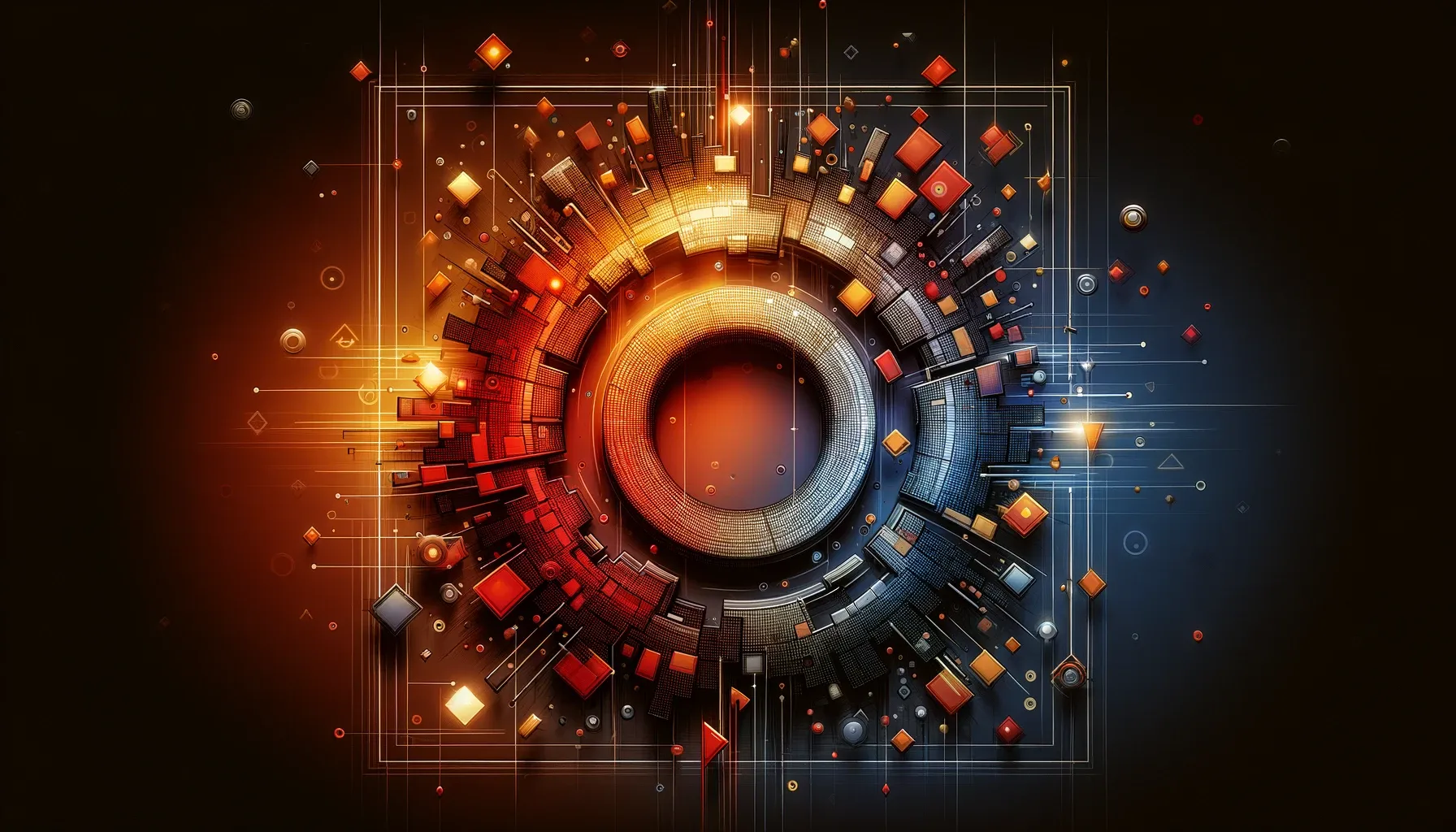
In the world of software development, few errors are as universally dreaded and as common as the null pointer exception (NPE). This sneaky bug can cause applications to crash unexpectedly and can be a major source of frustration for developers. Understanding what null pointer exceptions are, why they occur, and how to prevent them is crucial for anyone looking to write robust, error-free code.
What is a Null Pointer Exception?
A null pointer exception occurs when an application tries to use an object reference that has the null value. In simpler terms, it happens when your code attempts to access or modify the properties of an object that doesn't exist. Since there's no actual object to perform the operation on, the system throws an exception, often leading to application crashes or unpredictable behavior.
Why Do Null Pointer Exceptions Occur?
The root cause of null pointer exceptions is the improper handling of null values in code. These can sneak in through various avenues:
- Uninitialized Variables: Declaring an object reference without initializing it often leads to NPE, as the reference is null by default.
- Return Values: Methods that return an object can sometimes return null, especially if they fail to find or create the object they're supposed to return.
- External Data Sources: Data fetched from databases, files, or external services may contain null values, leading to NPE if not properly checked.
- Chaining Method Calls: Calling methods on an object returned by another method without checking for null can easily result in an NPE.
The Perils of Null Pointer Exceptions
The impact of null pointer exceptions goes beyond mere inconvenience. They can lead to several serious issues:
- Application Crashes: The most immediate and visible effect of an NPE is the sudden crash of an application, leading to a poor user experience and potential data loss.
- Security Vulnerabilities: In some cases, attackers can exploit NPEs to bypass certain checks or cause unexpected behavior, potentially leading to security breaches.
- Wasted Time and Resources: Identifying and fixing null pointer exceptions can be a time-consuming process, diverting valuable resources from feature development to bug fixing.
- Code Smell: Frequent null pointer exceptions are often a sign of poor code quality and design, indicating an over-reliance on nulls or a lack of proper error handling and validation mechanisms.
How to Prevent Null Pointer Exceptions
Preventing null pointer exceptions involves a combination of good coding practices and leveraging modern language features:
- Null Checks: Implement explicit checks for null values before using any object reference. This is a straightforward but crucial step in avoiding NPEs.
- Optional Objects: Some languages offer
Optional
or similar constructs that encapsulate an object that may or may not be null, forcing the developer to handle both cases explicitly. - Initialization: Ensure that all object references are properly initialized before use, either at the point of declaration or within a constructor.
- Use Annotations: Many development environments support annotations like
@NotNull
and@Nullable
to indicate whether a method or parameter can expect null values, helping developers catch potential null issues at compile time. - Better Error Handling: Implement comprehensive error handling and validation strategies to deal with null values gracefully, providing fallbacks or meaningful error messages instead of crashing.
Conclusion
While null pointer exceptions are a common pitfall in many programming languages, understanding their causes and implementing strategies to avoid them can greatly improve the stability and reliability of your code. By adopting best practices and making use of language features designed to handle null safely, developers can navigate the minefield of null pointer exceptions and build more robust applications.
Finding bugs like this can be difficult. If y0u would like our advice or have us take a look reach out to us at pelaghisoftware.com.